Introduction to C Programming Language
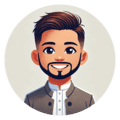
Full Stack Developer | SEO
The C programming language, developed in the early 1970s by Dennis Ritchie at Bell Labs, is one of the most powerful and widely used programming languages in the world. Known for its efficiency, flexibility, and performance, C serves as the foundation for many modern languages like C++, Java, and Python. Despite being over four decades old, C remains a popular choice for systeInm programming, embedded systems, game development, and performance-critical applications.
Whether you are a beginner in programming or an experienced developer, understanding C is essential for grasping the fundamentals of software development. In this article, we will explore the origins, features, syntax, and key components of the C programming language.
History of C
The C language was created as an evolution of the earlier languages, B and BCPL (Basic Combined Programming Language). Developed in 1972 by Dennis Ritchie, C was initially designed to write the UNIX operating system, which revolutionized computer science with its portability and efficiency.
Key milestones in C's history include:
1972: C language developed at Bell Labs.
1978: The first edition of "The C Programming Language" book by Brian Kernighan and Dennis Ritchie, also known as K&R C.
1989: The American National Standards Institute (ANSI) formalized C, leading to the creation of ANSI C or C89.
1999: Introduction of the C99 standard, which added several new features like inline functions, variable-length arrays, and new data types.
2011 and Beyond: Updates like C11 and C18 continue to make C relevant in modern programming.
Features of C
C has several features that contribute to its popularity:
Simple and Efficient: C is known for its simple syntax and efficient use of resources. It provides low-level access to memory, which makes it ideal for system programming.
Portability: C programs are highly portable, meaning code written on one machine can be easily compiled and run on another with minimal or no modifications.
Structured Language: C supports structured programming, which allows developers to write clean, modular, and maintainable code.
Rich Library: C comes with a vast standard library that provides a variety of built-in functions to perform common tasks, like input/output handling, string manipulation, and mathematical computations.
Low-Level Access: C allows direct access to hardware, making it suitable for writing system-level programs, device drivers, and embedded software.
Extensible: C programs can be easily extended by adding new functions and libraries, enabling developers to build upon existing code.
Basic Syntax of C
C programs are structured around functions, with the main()
function being the entry point of any C program. Here is a simple example of a "Hello, World!" program in C:
Explanation:
#include <stdio.h>
: This line includes the standard input-output library, which provides functions likeprintf()
for displaying output.int main()
: Themain()
function is where program execution begins. It returns an integer value, which represents the program's exit status.printf("Hello, World!\n")
: This line prints "Hello, World!" to the console. The\n
is a newline character.return 0;
: Thereturn
statement ends themain()
function and returns the value0
, indicating that the program executed successfully.
Key Components of C Programming
Variables and Data Types: C supports a variety of data types like
int
(integer),float
(floating-point),char
(character), anddouble
(double-precision floating-point). Variables are used to store data values of these types.Operators: C provides a wide range of operators, including arithmetic operators (
+
,-
,*
,/
), relational operators (==
,!=
,<
,>
), logical operators (&&
,||
,!
), and bitwise operators (&
,|
,^
).Control Structures: C uses control structures like
if
,else
,for
,while
, anddo-while
to control the flow of the program. These structures enable conditional execution and iteration.Functions: Functions are blocks of code that perform specific tasks and can be reused throughout the program. Functions help in organizing and modularizing code, making it easier to understand and maintain.
Pointers: Pointers are variables that store memory addresses. They provide powerful features for dynamic memory allocation, array handling, and working with data structures like linked lists and trees.
Arrays and Strings: Arrays are used to store multiple values of the same data type in a single variable. Strings are arrays of characters terminated by a null character (
'\0'
).Structures and Unions: Structures (
struct
) and unions (union
) are used to group different data types under a single name, allowing for the creation of complex data structures.Dynamic Memory Allocation: C provides functions like
malloc()
,calloc()
,realloc()
, andfree()
to dynamically allocate and deallocate memory at runtime.
Advantages of Learning C
Understanding of Core Programming Concepts: Learning C gives you a strong foundation in programming, as it covers core concepts like data types, memory management, and control structures.
Wide Applicability: C is widely used in various domains, from embedded systems and operating system development to game development and database management.
Foundation for Learning Other Languages: Understanding C makes it easier to learn other programming languages, especially those influenced by C, such as C++, Java, and JavaScript.
Performance and Control: C offers high performance and fine-grained control over system resources, making it an ideal choice for performance-critical applications.
Conclusion
he C programming language has withstood the test of time due to its simplicity, efficiency, and powerful features. Whether you are interested in systems programming, game development, or building high-performance applications, learning C is a worthwhile investment. By mastering C, you not only gain a deeper understanding of how computers work but also open up a world of possibilities in the software development field.
So, dive into the world of C programming, and start building your path to becoming an expert developer!