Introduction to C++ Programming Language
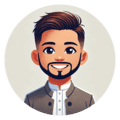
Full Stack Developer | SEO
C++ is a powerful, high-performance programming language that has been at the core of many modern software applications. Developed by Bjarne Stroustrup in the early 1980s as an extension of the C programming language, C++ introduced object-oriented programming (OOP) to provide a more structured and modular approach to software development. With its blend of low-level memory manipulation capabilities and high-level abstractions, C++ has become the language of choice for a wide range of applications, from operating systems and game engines to financial modeling and embedded systems.
In this article, we will explore the history, features, syntax, and applications of C++, as well as why learning C++ is essential for aspiring software developers.
History and Evolution of C++
C++ was created by Bjarne Stroustrup at Bell Labs in 1979 as an enhancement of the C programming language. Originally named "C with Classes," C++ aimed to add features that facilitated data abstraction, encapsulation, inheritance, and polymorphism—key concepts in Object-Oriented Programming (OOP). Stroustrup combined the efficiency and flexibility of C with these new paradigms, creating a language that could handle both low-level hardware manipulation and high-level software design.
Key points in the evolution of C++ include:
1983: The name "C++" was officially adopted, representing an increment (++) in the C language.
1985: The first edition of "The C++ Programming Language" book was published, providing the first complete guide to the language.
1989: The first standardized version, known as "C++ 2.0," was released, introducing multiple inheritance, abstract classes, and static member functions.
1998 (C++98): The ISO (International Organization for Standardization) published the first standard for C++, which added features like templates and the Standard Template Library (STL).
2011 (C++11): A significant update that introduced modern features such as auto type inference, range-based for loops, lambda expressions, smart pointers, and concurrency support.
2014 (C++14): Minor revisions to C++11, focusing on bug fixes and small enhancements.
2017 (C++17): Added new features such as
std::optional
,std::variant
, filesystem support, and improved compile-time programming.2020 (C++20): A major revision introducing concepts, ranges, coroutines, modules, and calendar/time utilities.
Future (C++23 and Beyond): Continuation of adding new features, improving usability, and maintaining backward compatibility.
Key Features of C++
C++ is known for its rich feature set that supports various programming paradigms, including procedural, object-oriented, and generic programming. Some of its key features are:
Object-Oriented Programming (OOP) Support: C++ was designed with OOP principles in mind, enabling the creation of reusable and modular code through classes, objects, inheritance, polymorphism, encapsulation, and abstraction.
Strong Type Checking: C++ has a robust type-checking mechanism that helps prevent errors by ensuring that variables are used consistently according to their data types.
Rich Standard Library: C++ comes with a comprehensive standard library, which includes the Standard Template Library (STL) for data structures and algorithms, input/output facilities, and various utility functions.
Compatibility with C: C++ retains most of C's syntax and functionality, allowing C code to be compiled and executed with minimal modification. This backward compatibility makes C++ a suitable choice for upgrading legacy C applications.
Low-Level Memory Manipulation: C++ allows direct manipulation of hardware and memory, which is crucial for systems programming, embedded systems, and performance-critical applications.
Templates and Generic Programming: C++ supports generic programming through templates, enabling the creation of flexible and reusable code. Templates allow functions and classes to operate with any data type without sacrificing performance.
Support for Multiple Paradigms: C++ supports multiple programming paradigms, such as procedural, object-oriented, and functional programming, making it a versatile language for different types of projects.
Modularity and Extensibility: C++ provides features like namespaces, classes, and functions that promote modularity and extensibility, allowing large programs to be organized and maintained effectively.
Basic Syntax and Structure
C++ programs are built around functions, with the main()
function serving as the entry point. Below is a simple "Hello, World!" program in C++:
Explanation:
#include <iostream>
: This line includes the input-output stream library, which provides functionalities likestd::cout
for output andstd::cin
for input.int main()
: Themain()
function is the entry point of any C++ program, where execution begins.std::cout
: Thecout
object, defined in thestd
namespace, is used to output data to the standard console.<<
: The insertion operator, used to send data to the output stream (cout
).std::endl
: This is used to insert a newline and flush the output buffer.return 0;
: Returns 0 to the operating system to indicate that the program executed successfully.
Object-Oriented Programming in C++
C++ is an object-oriented programming language, which means it allows developers to model real-world entities using objects and classes. Here are some key OOP concepts supported by C++:
Classes and Objects:
A class is a blueprint for creating objects. It defines data members (attributes) and member functions (methods) that operate on the data.
Inheritance: Allows a class to inherit attributes and methods from another class, promoting code reuse.
Polymorphism: Enables the same function to behave differently based on the object calling it, through method overriding or function overloading.
Encapsulation: Bundles the data and methods that manipulate the data within a single unit (class) and restricts direct access to some of the object's components.
Abstraction: Allows hiding complex implementation details while exposing only the essential parts.
Advanced Features of C++
Templates and the Standard Template Library (STL):
Templates: Allow the creation of generic classes and functions. They enable code reusability for different data types.
STL: A powerful library providing ready-to-use templates for data structures (like vectors, lists, stacks), algorithms (like sort, search), and iterators.
Memory Management:
Pointers and References: Provide low-level access to memory.
Dynamic Allocation:
new
anddelete
operators are used for dynamic memory allocation and deallocation, enabling more control over memory usage.
Exception Handling: C++ provides a robust mechanism for error handling using
try
,catch
, andthrow
blocks.Modern C++ Features:
Lambda Expressions: Anonymous functions that can capture variables from their surrounding context.
Smart Pointers:
unique_ptr
,shared_ptr
, andweak_ptr
help manage memory automatically, preventing memory leaks.Auto Keyword: Type inference for automatic type deduction.
Concurrency Support: Features for multi-threading and parallelism.
C++ Standard Libraries
C++ has a rich collection of standard libraries that provide a range of functionalities:
I/O Libraries:
iostream
for input/output operations.Container Libraries:
vector
,list
,map
, etc., for data storage and manipulation.Algorithm Libraries: Functions for searching, sorting, merging, and other algorithmic tasks.
Filesystem Library: Added in C++17 to handle file and directory operations.
Applications of C++
C++ is used in a variety of domains due to its flexibility, performance, and extensive feature set:
Game Development: Many game engines, like Unreal Engine, are built using C++ due to its high performance and ability to manage hardware resources effectively.
System Software: Operating systems like Windows, Linux, and macOS use C++ for core components.
Embedded Systems: C++ is widely used in the development of embedded systems, such as automotive software, IoT devices, and robotics.
Finance: High-frequency trading platforms and financial modeling tools often use C++ for its speed and low latency.
Web Browsers: Parts of popular browsers, such as Chrome and Firefox, are written in C++.
Real-Time Applications: C++ is ideal for applications that require real-time processing, such as simulations and virtual reality.
Advantages of Learning C++
Performance: C++ is known for its high performance, making it suitable for applications where speed and efficiency are critical.
Control Over System Resources: Direct access to memory and hardware allows for optimization in resource-constrained environments.
Foundation for Learning Other Languages: Understanding C++ provides a strong foundation for learning other languages, such as C, Java, and C#.
Understanding of Programming Concepts: Learning C++ gives a deep understanding of fundamental programming concepts like data structures, algorithms, memory management, and object-oriented design.
Challenges and Considerations
Complexity: C++ is hard to learn because of its complicated syntax and features.
Memory Management: Requires careful handling of memory to avoid leaks and undefined behavior.
Error-Prone: Due to its low-level capabilities, C++ can be error-prone if not used carefully.
Conclusion
C++ remains one of the most important and widely used programming languages in the world. Its combination of low-level programming capabilities with high-level abstractions makes it a versatile tool for a wide range of applications. Whether you are developing a high-performance game engine, an operating system, or a financial application, C++ provides the power and flexibility needed to build efficient and robust software. Learning C++ is an invaluable skill for any software developer, offering a strong foundation for mastering other programming languages and domains.