Node.js Introduction
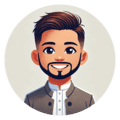
Full Stack Developer | SEO
Node.js is a cross-platform, server-side JavaScript runtime environment that allows you to run JavaScript outside the browser. Initially it was developed by Ryan Dahl 2009. It is compatible with major operating systems, including Windows, Linux, and macOS, and is widely used for building both backend and full-stack applications.
What is Node.js?
Node js is the runtime environment for JavaScript. Each browser has its own JavaScript engine that compiles JavaScript Code into machine code and executes.
Google Chrome uses the V8 Engine.
Mozilla Firefox uses the SpiderMonkey Engine.
Safari uses the JavaScriptCore (Nitro) Engine.
Opera uses V8 Engine
Microsoft Edge uses V8 Engine
Before the creation of Node.js, JavaScript was primarily used for client-side (browser) tasks, limited to running within these engines in the browser environment.
However, in 2009, Ryan Dahl introduced Node.js, which combined C++ with Chrome’s V8 Engine, allowing JavaScript to be executed outside the browser, on the server side. This marked a significant shift, as developers could now use JavaScript to build server-side applications, enhancing JavaScript's versatility.
Why Use Node Js?
Node.js has become a popular choice for server-side development for several reasons:
Single Language for Frontend and Backend: If you’re already using JavaScript on the frontend, you can use the same language on the backend with Node.js. This means you don’t need to learn a different language, simplifying full-stack development. It allows for code reusability between client and server sides and improves productivity.
High Performance: Node.js is built on Google Chrome’s V8 JavaScript engine, which executes JavaScript code. This ensures high performance and faster execution of code, making Node.js suitable for building scalable and high-performance applications.
Large and Active Community: Node.js has a large, vibrant, and active community. This means a wealth of resources, tutorials, and tools are available, and you can easily find support for any challenges you might face. It also means regular updates, enhancements, and a rich ecosystem.
Rich Ecosystem of Packages: Node.js has an extensive package ecosystem with npm (Node Package Manager), offering over a million packages that provide pre-built modules for almost any functionality you need. This reduces development time and effort significantly since you can integrate these modules into your project instead of building everything from scratch.
Scalability: Node.js is designed for scalability, using a single-threaded model with event looping. It can handle many concurrent connections with high throughput, which makes it suitable for applications that need to scale both vertically and horizontally.
JSON-Friendly: JavaScript Object Notation (JSON) is a popular data interchange format, and Node.js handles JSON exceptionally well. This makes it ideal for APIs and microservices that need to process JSON data.
Microservices Architecture: Node.js is a good fit for building microservices, as its lightweight nature and non-blocking architecture allow for decoupling and the creation of small, independently deployable services.
Difference between JavaScript and Node.js
Node js
Definition: Node.js is a runtime environment that allows JavaScript to be executed outside of the browser. It is built on Google Chrome’s V8 engine and enables the development of server-side applications.
Environment: Node.js provides an environment where JavaScript can be run directly on the operating system (Windows, Linux, macOS), outside of the browser.
Usage: Primarily used for server-side development and backend applications, such as APIs, web servers, and command-line tools. It can also be used in full-stack development, handling server and client logic.
No DOM Access: Node.js does not have access to the DOM or the window object because it is not running in a browser environment. Instead, it has its own global objects like global and process for server-side operations.
Modules: Node.js comes with a built-in module system that allows you to import and use various modules, such as fs (for file system operations), http (for creating web servers), and third-party modules like Express.js for building web applications.
Frameworks: Node.js frameworks like Express.js, Koa, and Nest.js are used for building robust backend services, APIs, and server-side applications.
Asynchronous and Non-blocking: Node.js uses an asynchronous, non-blocking I/O model that makes it highly efficient and suitable for applications that need to handle many concurrent connections, like real-time apps and chat systems.
NPM (Node Package Manager): Node.js has a vast ecosystem of libraries and packages available through npm, which makes it easy to include third-party modules in your applications and manage project dependencies.
JavaScript
Definition: JavaScript is a high-level, interpreted or JIT compiled programming language that is primarily adding user interaction in the websites. It is a core technology of the website, alongside HTML and CSS.
Environment: JavaScript code can only be executed within the context of a web browser (such as Chrome, Firefox, or Safari) without additional tools. Each browser has their own built-in JavaScript engines (like V8 in Chrome, SpiderMonkey in Firefox) to interpret and run JavaScript.
Usage: Primarily used for client-side scripting to enhance user interfaces and create interactive web pages. It allows for DOM manipulation, event handling, and animations.
DOM Access: JavaScript has access to the DOM (Document Object Model) and the window object, allowing JavaScript to manipulate HTML and CSS of the UI.
Frameworks: JavaScript frameworks and libraries like React js, Next js, Vue.js, and Angular are used to build complex and feature-rich frontend applications.
Limitations: JavaScript is restricted to the client-side in the browser environment, meaning it cannot interact directly with the file system, network, or OS-level functionalities.
In tables
Criteria | JavaScript | Node.js |
---|---|---|
Definition | High-level programming language for web development. | Runtime environment for executing JavaScript in the OS |
Environment | Runs in browser environments (Chrome, Firefox, etc.). | Runs directly on the operating system (Windows, Linux, macOS). |
Usage | Primarily used for client-side scripting. | Used for server-side and backend development. |
DOM Access | Has access to the DOM and window object. | No access to the DOM or window object. |
Primary Purpose | Enhancing user interfaces and creating interactive web pages. | Building server-side applications, APIs, and tools. |
Frameworks/Libraries | React.js, Vue.js, Angular for frontend development. | Express.js, Koa, Nest.js for backend development. |
Modules | Uses ES6 modules and libraries for frontend functionality. | Uses CommonJS modules; vast package ecosystem with npm. |
Global Objects | window, document (in browsers). | global, process, require, module (in Node.js). |
Execution Model | Single-threaded, event-driven in browsers. | Single-threaded, event-driven, non-blocking I/O model. |
Performance | Limited to browser performance optimizations. | High performance with the V8 engine, suitable for scalable apps. |
Asynchronous Support | Supports async/await for handling asynchronous code. | Built-in support for async operations; non-blocking I/O. |
NPM Support | Not available natively, relies on bundlers like Webpack. | Native support for npm, allowing easy integration of packages. |
Basic Example of Node.js Application
The smallest Node.js application for "Hello World" is:
This example demonstrates how to create a basic HTTP server using Node.js. The server listens on port 3000 and responds with "Hello World" to any incoming requests, showcasing Node.js's simplicity and power in handling HTTP requests.
Conclusion
Node.js is a cross-platform JavaScript runtime environment that allows execution outside the browser, initially developed by Ryan Dahl in 2009. It’s used for backend and full-stack applications due to its high performance, single language for both frontend and backend, large community, rich package ecosystem, and scalability. Node.js contrasts with traditional JavaScript, which runs in browsers, by enabling server-side development on various operating systems. It uses an asynchronous, non-blocking I/O model, making it highly efficient. Key benefits include handling JSON effortlessly, fitting microservices architecture, and providing robust frameworks like Express.js for backend services.